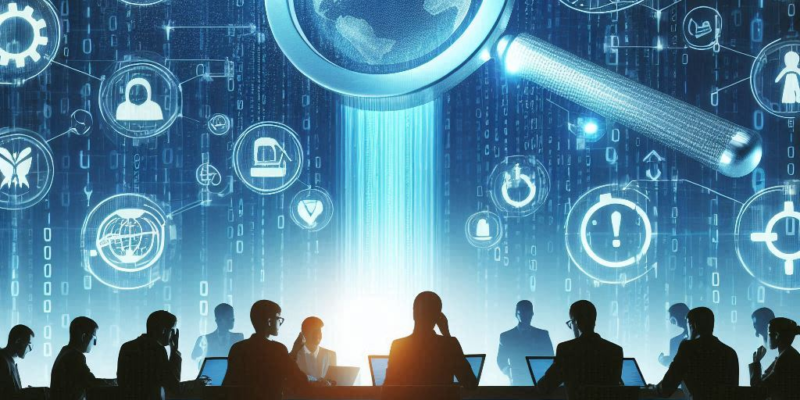
Computers face a similar challenge every day. The world we interact with – websites, games, even this text – is built on a foundation of ones and zeroes: binary code. But how do programs written for different processors, with unique instruction sets, communicate and work seamlessly? The answer lies in a fascinating process called binary translation.
Definition and Importance
Binary translation acts as a translator between different computer languages. It takes a program written in one set of instructions (source code) and converts it into another (target code) that a specific processor can understand. This ability to bridge the gap between instruction sets is crucial for modern computing. Without it, software wouldn’t run on different devices, emulators wouldn’t exist, and running older programs on newer machines would be impossible.
Understanding Binary Code
Before we dive into the wonders of binary translation, let’s establish a common ground: the language of computers, binary code.
The Binary Number System: A World of 0s and 1s
Imagine a world with only two numbers: 0 and 1. That’s the core principle of the binary system, a base-2 system compared to our everyday base-10 (decimal) system. In decimal, each digit’s position holds a value based on powers of 10. The rightmost digit is ones (10^0), the next is tens (10^1), and so on. Binary works similarly, but with powers of 2. The rightmost bit represents 2^0 (one), the next 2^1 (two), and so forth.
For example, the number 13 in decimal translates to 1101 in binary. Here’s the breakdown:
- 1 (leftmost digit) x 2^3 (eight) = 0 (doesn’t contribute to the final value)
- 0 (second digit) x 2^2 (four) = 0 (doesn’t contribute)
- 1 (third digit) x 2^1 (two) = 2
- 1 (rightmost digit) x 2^0 (one) = 1
Adding these positional values (0 + 0 + 2 + 1) gives us 13, the decimal equivalent.
Binary Digits: The Building Blocks (Bits)
The individual numbers in binary code (0 or 1) are called bits (short for binary digits). These bits act like tiny switches, either on (1) or off (0). This on/off state perfectly aligns with how computers operate, where electrical signals represent data. A high voltage can represent 1, and a low voltage can represent 0.
Eight bits grouped together form a byte, which is the fundamental unit of data storage in most computers. Each byte can represent 256 (2 raised to the power of 8) different values, allowing us to store letters, numbers, symbols, and even instructions for programs.
Binary in Action: Powering the Digital World
Binary code is the lifeblood of computers. From the instructions a processor executes to the images displayed on your screen, everything is ultimately represented by a series of 0s and 1s.
Hardware: In a computer’s memory, each memory location stores a byte of data, represented in binary. The CPU reads these bytes and performs operations based on the binary instructions it receives.
Software: Programs are written in high-level languages we understand (like Python or Java). These languages are then translated into machine code, which is essentially a set of binary instructions the computer can directly execute.
Understanding binary code isn’t essential for everyday computer use, but it sheds light on the fascinating inner workings of the digital world. With this foundation, we can now explore how different devices and programs communicate through the magic of binary translation.
The Science of Binary Translation
Unlocking the Language Barrier
Now that we’ve grasped the language of computers (binary code), let’s delve into how programs written for different instruction sets can understand each other. This is where binary translation comes in, acting as a skilled interpreter between processors.
Lost in Translation? Not with These Two Techniques
There are two main approaches to binary translation: static and dynamic.
Static Translation: This method happens before a program even runs. A translator program analyzes the source code (written in a high-level language) and converts it entirely into the target machine code (the specific instructions the target processor understands). This pre-translated code is then saved and executed directly, offering faster performance. A compiler is a classic example of a static translator.
Dynamic Translation (Just-in-Time – JIT): Here, translation occurs on the fly, during program execution. The program is initially stored in a high-level language or an intermediate format. As the program runs, the translator analyzes and converts only the sections of code being used at that moment. This approach offers greater flexibility but can incur a slight performance overhead compared to static translation. A Just-in-Time (JIT) compiler is a prime example of dynamic translation.
Behind the Scenes: From Human Language to Machine Code
Imagine you write a program in a language like Python. Here’s what happens behind the scenes:
High-Level Language to Assembly: A compiler translates your Python code into assembly language. Assembly is a low-level language that’s closer to machine code but still human-readable. It uses instructions specific to a particular processor family (e.g., x86, ARM).
Assembly to Machine Code: Here, an assembler takes the assembly code and converts it into the target processor’s specific binary instructions (machine code). This code is what the CPU directly understands and executes.
In Binary Translation: The process can occur at either the assembly or machine code level. A static translator might convert the entire assembly code to the target machine code upfront. Conversely, a JIT translator might translate blocks of machine code only as the program needs them.
Algorithms and the Art of Efficiency
Binary translation relies on clever algorithms to analyze and convert code efficiently. Some common algorithms include:
Instruction Set Mapping: This involves finding equivalent instructions in the target processor’s set that achieve the same functionality as the source instructions.
Register Allocation: Optimizing how data is stored in the processor’s temporary storage locations (registers) can significantly impact performance.
Dead Code Elimination: Identifying and removing unnecessary code sections from the source code can streamline the translated code.
Techniques like these not only ensure accurate translation but also optimize the resulting code for speed and efficiency on the target processor.
By understanding these concepts, we gain a deeper appreciation for the complex dance that allows programs from different worlds to coexist and function seamlessly on our devices.
How Binary Translation Works
A Step-by-Step Journey
Now, let’s dissect the fascinating process of binary translation step-by-step. We’ll use an example to illustrate the concepts.
Imagine you have a program written in Python that calculates the area of a circle (source code).
Preparation (Optional): Depending on the translation type:
- Static: The entire Python code might be pre-processed to gather information about variables, functions, and dependencies.
- Dynamic: The initial translation might convert the Python code to an intermediate format (like bytecode) suitable for further on-the-fly translation.
Analysis: The translator examines the source code (assembly or machine code) to understand the instructions and data involved.
Mapping: The core of translation. Here, the translator finds equivalent instructions in the target processor’s instruction set that achieve the same results as the source instructions. For example, an “add” instruction in the source code might be translated to a specific “add” instruction supported by the target processor.
Example: Let’s say our Python program has the line area = 3.14 * radius * radius. During translation, this might be converted to assembly instructions for calculating the product and assigning the result to a variable. These assembly instructions would then be translated into the target processor’s specific machine code for multiplication and assignment operations.
Building the Bridge
Several key tools and technologies play a crucial role in binary translation:
Compilers and Assemblers: These traditional tools can be considered static binary translators, converting code from high-level languages to assembly or machine code for specific processors.
Dynamic Transpilation Tools: Frameworks like GCC’s GIMPLE and LLVM’s Intermediate Representation (IR) allow for dynamic translation by providing a common intermediate format that can be translated to various target architectures.
Hardware-Assisted Binary Translation (HBT): Modern processors sometimes include built-in features to assist with binary translation on the fly, further improving performance.
Challenges and the Quest for Solutions
Despite its strengths, binary translation faces some challenges:
Complexity of Modern Processors: Modern processors have complex instruction sets and features that can be challenging to translate efficiently.
Security Concerns: Malicious code hidden within the source program might be more difficult to detect during translation.
Researchers are addressing these challenges by developing more sophisticated analysis techniques and security checks during the translation process. Additionally, collaboration between hardware and software developers can lead to more efficient translation capabilities within processors themselves.
By understanding the intricacies of binary translation, we gain a deeper appreciation for the complex world that keeps our devices running smoothly. As technology evolves, binary translation will continue to play a vital role in ensuring seamless communication and compatibility between programs and processors, paving the way for a more interconnected digital future.
Why Binary Translation Matters
Efficiency, Performance, and Beyond
Binary translation offers a powerful tool, but it’s not without its trade-offs. Let’s delve into the impact of binary translation on various aspects of computing.
Efficiency and Performance:
Double-edged Sword: The translation process itself introduces some overhead. However, by optimizing the translated code for the target architecture, binary translation can sometimes lead to performance improvements. For example, a JIT compiler can translate code sections frequently used in a program, resulting in faster execution on subsequent runs.
Real-World Example: Translating legacy software written for older processors to run on modern machines can breathe new life into them, utilizing the increased processing power more efficiently.
Compatibility and Portability:
Bridging the Gap: Binary translation allows software written for one processor to run on another, enhancing compatibility. This is especially crucial for running older programs on newer machines or for emulating different architectures.
Cross-Platform Development: By enabling code to run on various platforms, binary translation streamlines the development process for cross-platform applications. Developers can write code once and potentially translate it for different target devices.
Security Implications:
Double Agent: Binary translation can offer security benefits. By analyzing code during translation, malicious instructions can potentially be identified and removed.
Hidden Threat: Conversely, malicious code embedded within the source program might be more difficult to detect during translation, posing a potential security risk. This highlights the importance of secure coding practices and careful analysis of the source code before translation.
Where Binary Translation Shines
Binary translation finds applications in various domains:
Operating Systems: Some operating systems utilize binary translation to improve performance by translating frequently used code sections to a more optimized form for the specific processor.
Software Development: Tools like emulators and virtual machines rely heavily on binary translation to run programs designed for different architectures within their simulated environments. Popular examples include emulators for running mobile apps on computers (e.g., BlueStacks) and virtual machines for running different operating systems on a single machine (e.g., VirtualBox).
In conclusion, binary translation acts as a bridge between different computing worlds. By understanding its impact on efficiency, performance, security, and compatibility, we gain a deeper appreciation for its role in shaping the ever-evolving digital landscape.
Conclusion
Binary translation has emerged as a critical force in the world of computing. It acts as a skilled translator, enabling programs written for one processor architecture to seamlessly run on another. This seemingly magical feat unlocks a treasure trove of benefits:
- Compatibility: Binary translation bridges the gap between different systems, allowing us to run older programs on newer machines and facilitating cross-platform development.
- Performance: While the translation process itself adds some overhead, optimization techniques can sometimes lead to performance improvements by tailoring the code for the target processor.
- Security: The ability to analyze code during translation offers a potential layer of security by identifying and removing malicious instructions.
The story of binary translation is one of continuous evolution. As processors become more complex and the digital landscape expands, this technology will undoubtedly play an even greater role in ensuring seamless communication and compatibility between programs and devices. This understanding is just the first step. If you’re curious to delve deeper, explore the fascinating world of compilers, assemblers, and the intricacies of processor architectures. The journey into the heart of binary translation awaits